[TypeScript]Personal Notes
keywords: TypeScript, JavaScript Engine, Nodejs, Chrome, V8, Notes
Environment Setup for TypeScript
How to update TypeScript to latest version with npm
npm install -g typescript@latest
or
yarn global add typescript@latest // if you use yarn package manager
Origin:
https://stackoverflow.com/a/42155457/1645289
Setup compilation environment for Visual Studio Code
1, Install compiler (tsc) of TypeScript:
npm install typescript -g
2, Create a directory as your project, named myproj
.
3, Create a TypeScript source file under myproj
, named hello.ts
, and print a message in console:
let message: string = 'Hello';
console.log(message);
then compile source with command:
tsc hello.ts
4, Address to directory myproj
in command, then generate tsconfig.json
using command:
tsc --init
Reference:
https://zhuanlan.zhihu.com/p/115675528
5, Go to your project directory and excute commands:
npm install typescript --save
npm install ts-node --save-dev
Reference:
https://cloud.tencent.com/developer/article/1760857
If you got error:
Error: Cannot find module 'typescript'
Go to your project directory and link typescript to the project:
cd myproj
npm link typescript
6, Run npx ts-node
to test your source code:
npx ts-node hello.ts
Reference:
https://stackoverflow.com/a/65508481/1645289
7, Install extension TypeScript Debugger
, then click Run
-> Add Configuration
-> select TS Debug
:
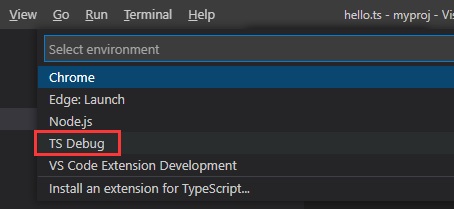
8, Open directory myproj
in Visual Studio Code, then press F5
or click Run
-> Start Debugging
.
Issue:
If you get error on Windows while Start Debugging
:
tsc.ps1 cannot be loaded because running scripts is disabled on this system
Excute command using PowerShell as administrator, and select option A(ll)
:
Set-ExecutionPolicy -ExecutionPolicy RemoteSigned
Reference:
https://stackoverflow.com/a/58801137/1645289
Now you would run debugging successfully.
Setup compilation environment for Intellij IDEA
Do first five above-side steps, then compile TypeScript source by clicking lable which was in the bottom of editor viewport, then debug your code.
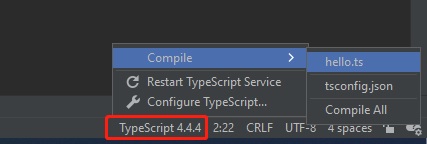
TypeScript plugin is only usable for Intellij IDEA Ultimate Edition
and WebStorm.
IntelliJ IDEA Plugins
IntelliJ IDEA (and WebStorm…) plugin to support ‘TypeScript’ as a run configuration
https://github.com/bluelovers/idea-run-typescript
Issues
Issue 2:
If you get error while Start Debugging
:
D:\SDKs\nodejs\node.exe -r ts-node/register .vscode\launch.json
SyntaxError: D:\myproj\.vscode\launch.json: Unexpected token / in JSON at position 7
at parse (<anonymous>)
at Object.Module._extensions..json (internal/modules/cjs/loader.js:1128:22)
at Module.load (internal/modules/cjs/loader.js:950:32)
at Function.Module._load (internal/modules/cjs/loader.js:790:12)
at Function.executeUserEntryPoint [as runMain] (internal/modules/run_main.js:76:12)
at internal/main/run_main_module.js:17:47
Process exited with code 1
Or:
Debugger attached.
Waiting for the debugger to disconnect...
d:\myproj\hello.ts:1
let message: string = 'Hello World';
^
SyntaxError: Unexpected token ':'
at wrapSafe (internal/modules/cjs/loader.js:1001:16)
at Module._compile (internal/modules/cjs/loader.js:1049:27)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:1114:10)
at Module.load (internal/modules/cjs/loader.js:950:32)
at Function.Module._load (internal/modules/cjs/loader.js:790:12)
at Function.executeUserEntryPoint [as runMain] (internal/modules/run_main.js:76:12)
at internal/main/run_main_module.js:17:47
Process exited with code 1
It’s the bug of extentsion TypeScript Debugger
of Visual Studio Code.
https://github.com/microsoft/vscode/issues/45616
Recommended to use IntelliJ IDEA intead of Visual Studio Code.
Common API
How to print call stack when error occurs.
try {
// Code throwing an exception
} catch(e) {
console.log(e.stack);
}
Origin:
https://stackoverflow.com/a/4200200/1645289
string format
var text = "blah blah";
var strTest = `This is a ${text}`;
console.log(strTest);
String interpolation in Typescript, replacing ‘placeholders’ with variables
https://stackoverflow.com/a/52196435/1645289
Declare variables and export them
export namespace RoleCreationDefine
{
const Test01: string = "Neil Wang";
}
Declare enum and export them
export const enum ESexType {
None,
Male,
Female,
}
Declare dictionary and export it
We can try with built-in typescript advanced type called Record<K, T>
:
interface MyInterface {
data: Record<string, Item>;
}
Put everything together here
interface Item {
id: string;
name: string;
}
interface MyInterface {
data: Record<string, Item>;
}
export const obj: MyInterface = {
data: {
"123": { id: "123", name: "something" }
}
};
Origin: Dictionary type in TypeScript
https://stackoverflow.com/a/56353483/1645289
Frameworks & Libraries
V8 C++ Wrapping
Getting started with embedding V8
https://v8.dev/docs/embed
Bind C++ functions and classes into V8 JavaScript engine
https://github.com/pmed/v8pp
Lua
TypeScriptToLua. Typescript to lua transpiler.
https://github.com/TypeScriptToLua/TypeScriptToLua
GPU Rendering
A GPU-accelerated computing library for physics simulations and other mathematical calculations
https://github.com/amandaghassaei/gpu-io
NPM
Issues
Error on running npm install
:
error code EPERM
error syscall open
error path D:\GamesLibrary\EpicGamesStore\UE_4.27\Engine\Source\Programs\PixelStreaming\WebServers\SignallingWebServer\package-lock.json
error errno -4048
error Error: EPERM: operation not permitted, open 'D:\GamesLibrary\EpicGamesStore\UE_4.27\Engine\Source\Programs\PixelStreaming\WebServers\SignallingWebServer\package-lock.json'
error [Error: EPERM: operation not permitted, open 'D:\GamesLibrary\EpicGamesStore\UE_4.27\Engine\Source\Programs\PixelStreaming\WebServers\SignallingWebServer\package-lock.json'] {
error errno: -4048,
error code: 'EPERM',
error syscall: 'open',
error path: 'D:\\GamesLibrary\\EpicGamesStore\\UE_4.27\\Engine\\Source\\Programs\\PixelStreaming\\WebServers\\SignallingWebServer\\package-lock.json'
error }
error The operation was rejected by your operating system.
error It's possible that the file was already in use (by a text editor or antivirus),
error or that you lack permissions to access it.
error
error If you believe this might be a permissions issue, please double-check the
error permissions of the file and its containing directories, or try running
error the command again as root/Administrator.
verbose exit -4048
Solution:
npm cache clean --force
npm install -g npm@latest --force
Origin:
https://stackoverflow.com/a/59045453/1645289
References
Fragments
Mastering mapped types in TypeScript
https://blog.logrocket.com/mastering-mapped-types-typescript/
Performance
Why is TypeScript surpassing Python?
https://blog.logrocket.com/why-is-typescript-surpassing-python/
Books
Mastering TypeScript: Build enterprise-ready, modular web applications using TypeScript 4 and modern frameworks, 4th Edition 4th Edition (April 23, 2021)
https://www.amazon.com/Mastering-TypeScript-enterprise-ready-applications-frameworks-ebook/dp/B091D7P533/
“As a child I felt myself to be alone, and I am still, because I know things and must hint at things which others apparently know nothing of, and for the most part do not want to know.” ― Carl Gustav Jung, Memories, Dreams, Reflections